NumPy & Pandas สำหรับผู้เริ่มต้นงาน Data Science (Ep.1/2)
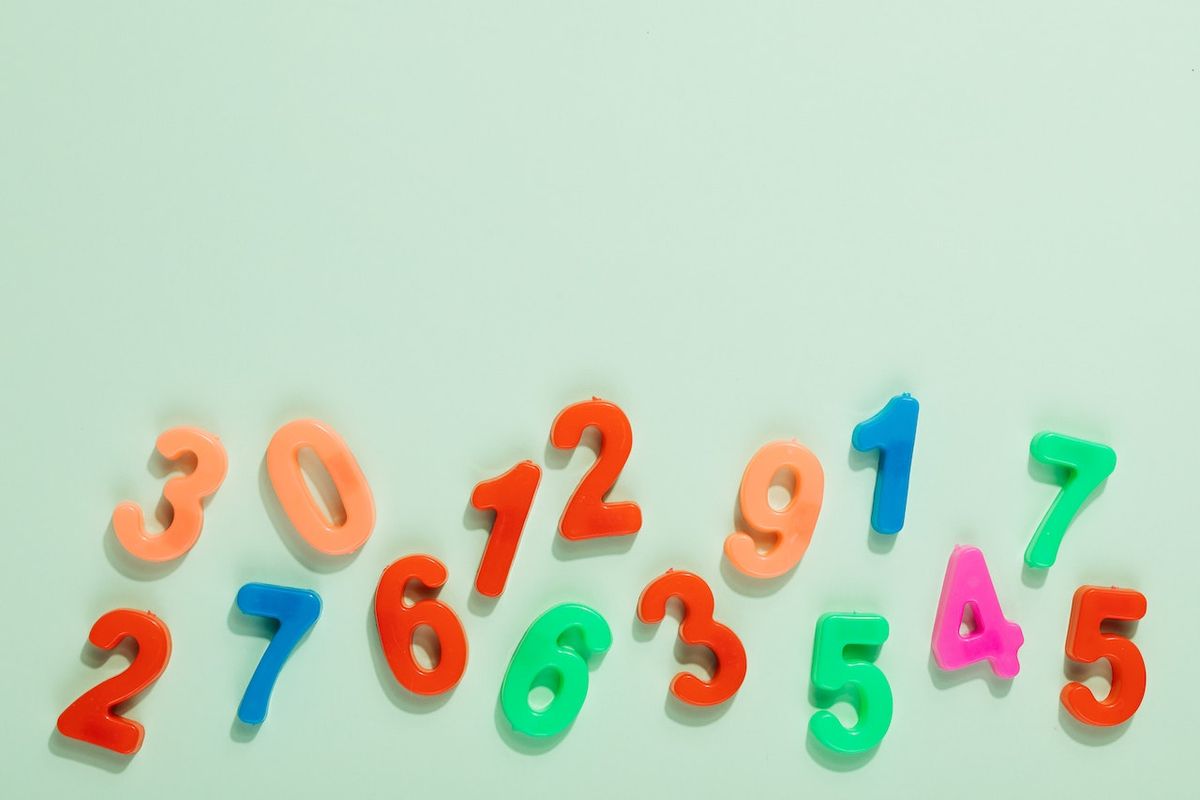
Python เป็นภาษาที่ได้รับความนิยมมาก ในงาน Data Science เนื่องจากความง่าย รวมไปถึง มี Community ขนาดใหญ่ และ Open Source Libraries ที่พร้อมใช้งานจำนวนมาก เช่น การจัดการข้อมูล การประยุกต์ใช้ Machine Learning & Deep Learning Models สำหรับผู้เริ่มต้นในงานด้าน Data Science มี Python Libraries ที่มีประโยชน์ และ ควรทำความคุ้นเคย คือ NumPy และ Pandas
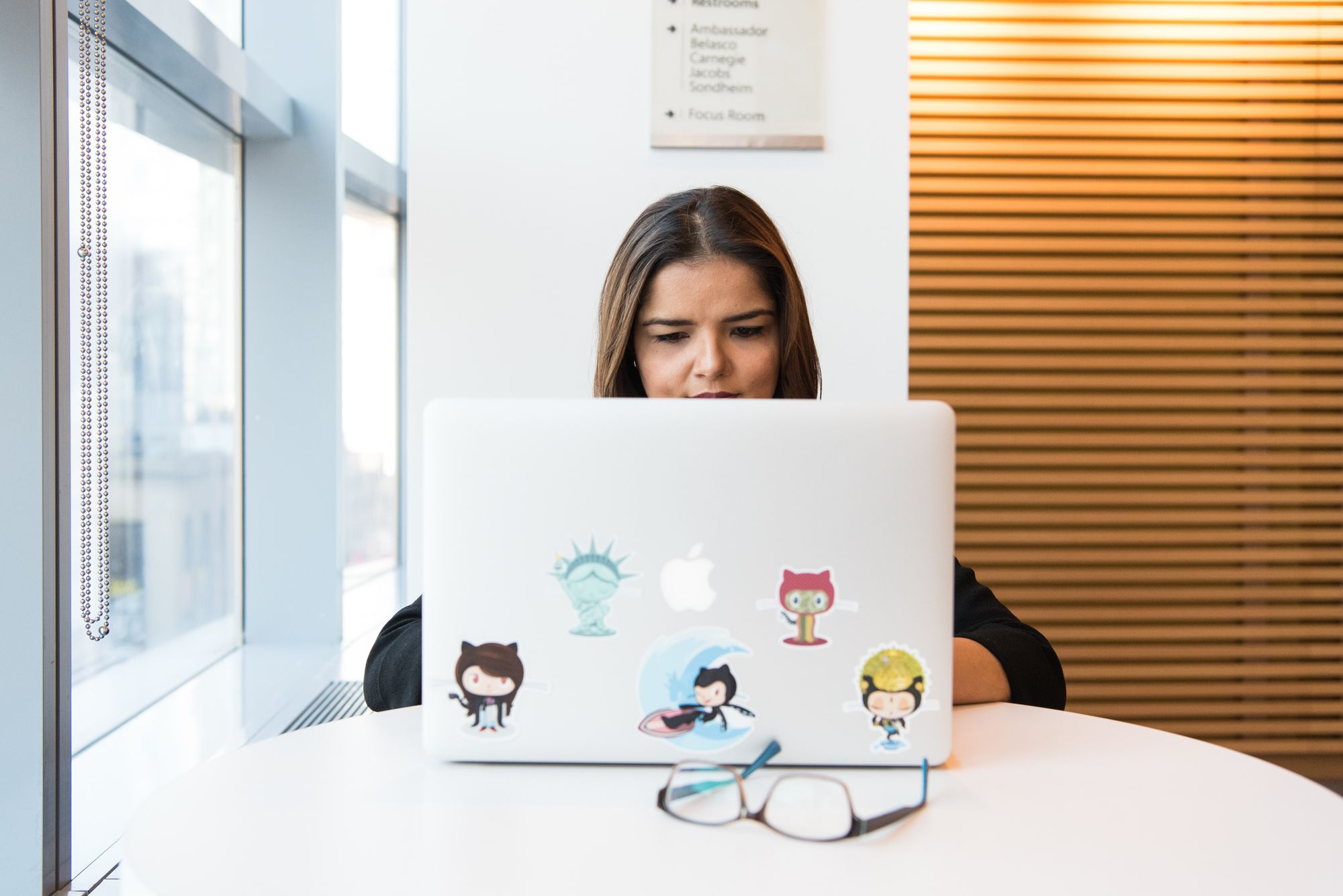
ใน Blog นี้ จะพูดถึงทั้ง 2 Libraries นี้
NumPy คืออะไร?
NumPy ย่อจาก Numerical Python ใช้เพื่อดำเนินการคำนวณ Arrays และ Matrices ได้อย่างมีประสิทธิภาพ ซึ่งเป็นเบื้องหลังของ Machine Learning Models โดยที่ Building Block ของ NumPy คือ Array ซึ่งเป็น Data Structure ที่คล้ายกับ List แต่มีความแตกต่างตรงที่มีฟังก์ชันทางคณิตศาสตร์จำนวนมาก กล่าวอีกนัยหนึ่ง NumPy Array เป็น Multi-dimensional Arrays
import numpy as np
A = [[1,2,3],[4,5,6],[7,8,9]]
np_arr = np.array(A)
np_arr
array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
เห็นว่าแตกต่างจาก List เราสามารถมองเห็น Matrix 3 x 3 ที่มีการเยื้องระหว่างแต่ละแถว นอกจากนี้ NumPy ยังมีมากกว่า 40 Functions ในการสร้าง Array
ในการสร้าง Array ที่เป็นเลขศูนย์ มี Functions เรียกว่า np.zeros ซึ่งเราเพียงแคระบุ Shape ที่ต้องการ
zeros_arr = np.zeros((2,3))
zeros_arr
array([[0., 0., 0.],
[0., 0., 0.]]
ในทำนองเดียวกัน สามารถสร้าง Array ที่เป็นเลขหนึ่งได้ ดังนี้
ones_arr = np.ones((3,4))
ones_arr
array([[1., 1., 1., 1.],
[1., 1., 1., 1.],
[1., 1., 1., 1.]])
สามารถสร้าง Identity Matrix ได้ ดังนี้
identity_arr = np.identity(3)
identity_arr
array([[1., 0., 0.],
[0., 1., 0.],
[0., 0., 1.]])
สามารถสร้าง Random Array ได้ โดยระบุ Shape ที่ต้องการ ดังนี้
random_arr = np.random.rand(2,3)
random_arr
array([[0.3186192 , 0.74390672, 0.660807 ],
[0.76079914, 0.34912427, 0.49333967]])
สามารถสร้าง Random Array ได้ และ ครั้งนี้ระบุเป็น Standard Normal Distribution
randn_arr = np.random.randn(10)
randn_arr
array([ 1.0333856 , 0.47816013, 0.20911559, -0.88532479, 1.01613286,
-0.26363197, -0.98441529, 0.54770656, -1.27739113, -0.41829642])
สามารถสร้าง Random Array ได้ โดยระบุค่าต่ำสุด สูงสุด และ จำนวนที่ต้องการ
randint_arr = np.random.randint(1,10,15)
randint_arr
array([8, 1, 6, 6, 5, 1, 9, 4, 1, 4, 3, 8, 8, 9, 3])
Index และ Slicing
นอกจาก Function ในการสร้าง Array แล้ว NumPy ยังสามารถเลือก Elements จาก Array ได้ โดยใช้ [..] ดังนี้
A = np.array([[1,2,3],[4,5,6]])
A[0]
array([1, 2, 3])
ต้องการเลือก Element ในแถวที่ 2 คอลัมน์ที่ 3
print(A[1,2])
6
อีกทางเลือกหนึ่งคือใช้ A[1][2] แต่จะไม่มีประสิทธิภาพเนื่องจากสร้าง Array แถวที่ 2 ก่อน จากนั้นจึงเลือก Element จากแถวนั้น
นอกจากนี้ สามารถ Slice Matrix โดยระบุ start:stop:step ในวงเล็บ โดยที่ Stop Index จะไม่ถูกรวมอยู่ด้วย เช่น เลือกแถวที่ 2 แต่ใช้แค่สอง Elements แรก
print(A[1,0:2])
[4 5]
อีกหากต้องการเลือกทุกแถว สำหรับคอลัมน์ที่ 2 ทำได้ดังนี้
print(A[:,1])
[2 5]
นอกจากการทำ Integer Array Indexing แล้ว ยังสามารถทำ Boolean Array Indexing ในการเลือก Element จาก Array ได้ด้วย กรณีต้องการ Elements ที่ตรงตามเงื่อนไขต่อไปนี้
A>4
array([[False, False, False],
[False, True, True]])
หากทำการ Filter Array โดย Condition ดังกล่าว ผลลัพธ์จะได้เป็น Elements จริงจาก Array
A[A>4]
array([5, 6])
Array Manipulation
ในการทำงานโครงการ Data Science บ่อยครั้ง จะเปลี่ยน Shape ของ Array โดยที่ข้อมูลยังคงเดิม เช่น Array เริ่มต้น ที่มี Shape 2x3
A = np.array([[1,2,3],[4,5,6]])
print(A)
print('Shape of Array: ',A.shape)
[[1 2 3]
[4 5 6]]
Shape of Array: (2, 3)
ทำการเปลี่ยนเป็น Array ที่มี Shape 3x2
A = A.reshape(3,2)
print(A)
print('Shape of Array: ',A.shape)
[[1 2]
[3 4]
[5 6]]
Shape of Array: (3, 2)
อีกกรณีหนึ่ง คือ การเปลี่ยน Array หลายมิติให้เป็นมิติเดียว ทำได้โดยระบุ -1 เป็น Shape
A = A.reshape(-1)
print(A)
print('Shape of Array: ',A.shape)
[1 2 3 4 5 6]
Shape of Array: (6,)
สามารถทำ Transpose ได้
A = np.array([[1,2,3,4,5,6]])
print('Before transpose shape of Array: ',A.shape)
A = A.T
print(A)
print('After transpose shape of Array: ',A.shape)
Before transpose shape of Array: (1, 6)
[[1]
[2]
[3]
[4]
[5]
[6]]
After transpose shape of Array: (6, 1)
หรือ ทำนองเดียวกัน สามารถใช้ np.transpose(A)
Array Multiplication
ในการสร้าง Machine Learning Algorithms ตั้งแต่เริ่มต้น อาจต้องคำนวณผลคูณ Matrix ของ 2 Arrays ซึ่งทำได้โดยใช้ np.matmul เมื่อ Array มีมากกว่า 1 มิติ (ในแบบสั้นกว่า คือ @)
A1 = np.array([[1,2,3],[4,5,6]])
A2 = np.array([[1,2],[3,4],[5,6]])
print('Shape of Array A1: ',A1.shape)
print('Shape of Array A2: ',A2.shape)
A3 = np.matmul(A1,A2)
# A3 = A1 @ A2
print(A3)
print('Shape of Array A3: ',A3.shape)
Shape of Array A1: (2, 3)
Shape of Array A2: (3, 2)
[[22 28]
[49 64]]
Shape of Array A3: (2, 2)
หรือ หากต้องการคูณ Matrix ด้วย Scalar ทำได้โดยใช้ np.dot (ในแบบสั้นกว่า คือ *)
A1 = np.array([[1,2,3],[4,5,6]])
A3 = np.dot(A1,2)
# A3 = A1 * 2
print(A3)
print('Shape of Array A3: ',A3.shape)
Mathematical Functions
NumPy มี Functions ทางคณิตศาสตร์ที่หลากหลาย เช่น ตรีโกณมิติ การปัดเศษ เลขชี้กำลัง ลอการิทึม และอื่นๆ สามารถดูรายละเอียดทั้งหมดได้ ที่นี่
ในที่นี้ จะแสดงตัวอย่าง เลขชี้กำลัง (Exponential) และ ลอการิทึมธรรมชาติ (Natural Logarithm)
A = np.array([[1,2],[3,4]])
print(np.exp(A))
[[ 2.71828183 7.3890561 ]
[20.08553692 54.59815003]]
หรือ การหาค่า Min / Max ใน Array
A = np.array([[1,2],[3,4]])
print(np.min(A),np.max(A))
1 4
หรือ การหาค่า Square Root ของแต่ละ Element ใน Array
A = np.array([[1,2],[3,4]])
print(np.sqrt(A))
[[1. 1.41421356]
[1.73205081 2. ]]
******
มีต่อ Ep.2 (ตอนจบ) อ่านได้ที่นี่ ->https://www.nerd-data.com/numpy-pandas-ep2/
******
ข้อมูลอ้างอิง - https://www.kdnuggets.com/introduction-to-numpy-and-pandas