7 Advanced Plots using Plotly
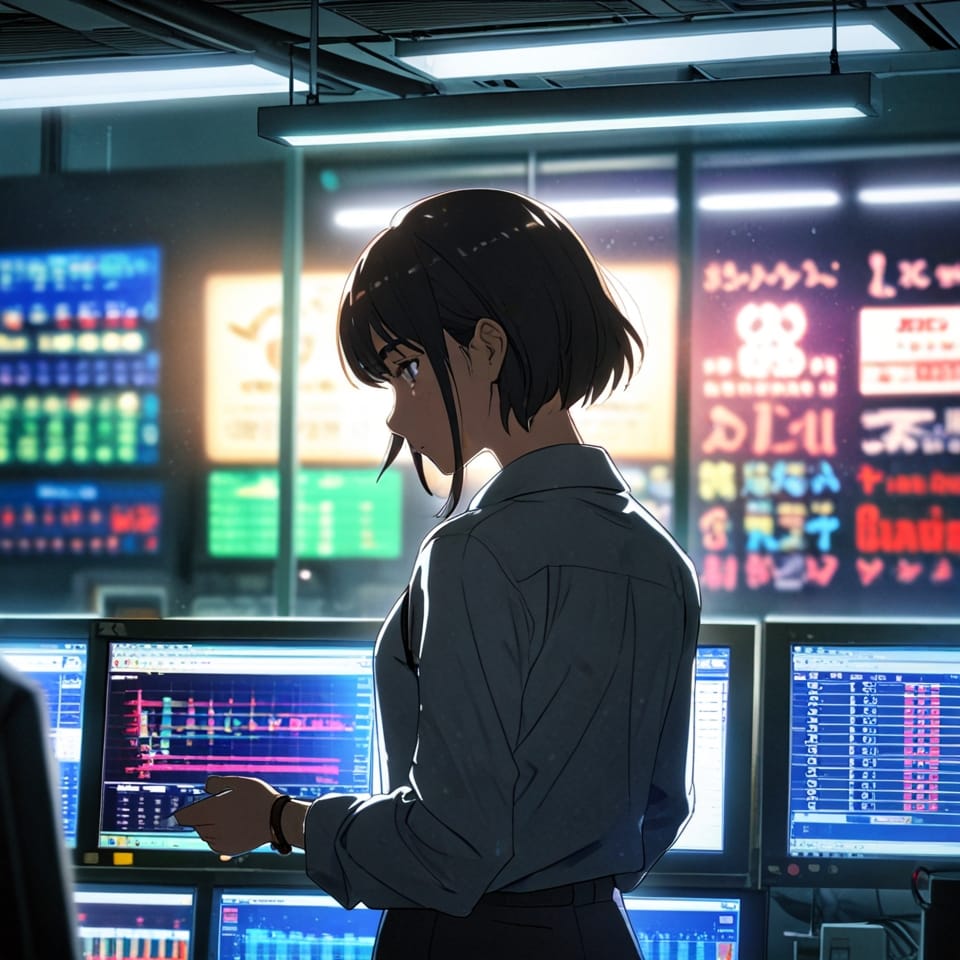
ตัวอย่าง Advanced Plots ที่สามารถทำได้โดยใช้ Plotly library ดังนี้
1) Sankey Diagram:
เป็นแผนภูมิการไหลที่แสดงการกระจายของทรัพยากรหรือปริมาณระหว่างหมวดหมู่ต่างๆ เหมาะสำหรับการแสดงระบบหรือกระบวนการที่ซับซ้อน หรือ ประยุกต์ใช้กับ Customer Journey ได้ ดูว่าขั้นตอนใดมีการ Drop off จำนวนมาก
2) Radar Chart:
หรือที่เรียกว่าแผนภูมิใยแมงมุมหรือแผนภูมิดาว มีประโยชน์ในการเปรียบเทียบตัวแปรเชิงปริมาณหลายตัว มักใช้ในการวิเคราะห์กีฬา การวิเคราะห์ประสิทธิภาพ และการประเมินทักษะ
3) Treemap:
เป็นวิธีที่มีประสิทธภาพในการแสดงข้อมูลแบบลำดับชั้นโดยใช้สี่เหลี่ยมผืนผ้าซ้อนกัน มักใช้ในการแสดงระบบไฟล์ โครงสร้างองค์กร หรือหมวดหมู่ผลิตภัณฑ์
4) Sunburst Diagram:
เหมาะสำหรับแสดงข้อมูลแบบลำดับชั้นในรูปแบบวงกลม คล้ายกับแผนภูมิต้นไม้แต่อยู่ในรูปแบบรัศมี
5) Parallel Coordinates Plot:
แผนภูมิประเภทนี้มีประโยชน์ในการแสดงและวิเคราะห์ข้อมูลหลายตัวแปร
6) 3D Surface Plot:
แผนภูมิพื้นผิว 3 มิติเหมาะสำหรับการแสดงข้อมูลสามมิติหรือฟังก์ชันทางคณิตศาสตร์
7) Violin Plot:
แผนภูมิไวโอลินมีประโยชน์ในการแสดงการกระจายของข้อมูลในหมวดหมู่ต่างๆ
ตัวอย่าง Python 🐍 code
import plotly.graph_objects as go
import pandas as pd
import numpy as np
# Sankey Diagram
fig_sankey = go.Figure(data=[go.Sankey(
node = dict(
pad = 15,
thickness = 20,
line = dict(color = "black", width = 0.5),
label = ["A", "B", "C", "D", "E", "F"],
color = "blue"
),
link = dict(
source = [0, 1, 0, 2, 3, 3],
target = [2, 3, 3, 4, 4, 5],
value = [8, 4, 2, 8, 4, 2]
))])
fig_sankey.update_layout(title_text="Basic Sankey Diagram", font_size=10)
# Radar Chart
categories = ['Processing Speed','Logical Reasoning','Verbal Ability',
'Spatial Reasoning','Memory','Mathematical Ability']
fig_radar = go.Figure()
fig_radar.add_trace(go.Scatterpolar(
r=[5, 4, 3, 3, 4, 5],
theta=categories,
fill='toself',
name='Person A'
))
fig_radar.add_trace(go.Scatterpolar(
r=[4, 3, 5, 2, 4, 3],
theta=categories,
fill='toself',
name='Person B'
))
fig_radar.update_layout(
polar=dict(radialaxis=dict(visible=True, range=[0, 5])),
showlegend=True
)
# Treemap
fig_treemap = go.Figure(go.Treemap(
labels = ["Eve", "Cain", "Seth", "Enos", "Noam", "Abel", "Awan", "Enoch", "Azura"],
parents = ["", "Eve", "Eve", "Seth", "Seth", "Eve", "Eve", "Awan", "Eve" ],
values = [10, 14, 12, 10, 2, 6, 6, 4, 4],
))
fig_treemap.update_layout(margin = dict(t=50, l=25, r=25, b=25))
# Sunburst Chart
fig_sunburst = go.Figure(go.Sunburst(
labels=["Eve", "Cain", "Seth", "Enos", "Noam", "Abel", "Awan", "Enoch", "Azura"],
parents=["", "Eve", "Eve", "Seth", "Seth", "Eve", "Eve", "Awan", "Eve"],
values=[10, 14, 12, 10, 2, 6, 6, 4, 4],
))
fig_sunburst.update_layout(margin = dict(t=0, l=0, r=0, b=0))
# Parallel Coordinates Plot
fig_parcoords = go.Figure(data=
go.Parcoords(
line_color='blue',
dimensions = list([
dict(range = [1,5],
constraintrange = [1,2], # change this range by dragging the pink line
label = 'A', values = [1,4]),
dict(range = [1.5,5],
tickvals = [1.5,3,4.5],
label = 'B', values = [3,1.5]),
dict(range = [1,5],
tickvals = [1,2,4,5],
label = 'C', values = [2,4],
ticktext = ['text 1', 'text 2', 'text 3', 'text 4']),
dict(range = [1,5],
label = 'D', values = [4,2])
])
)
)
# 3D Surface Plot
x = np.outer(np.linspace(-2, 2, 30), np.ones(30))
y = x.copy().T
z = np.cos(x ** 2 + y ** 2)
fig_surface = go.Figure(data=[go.Surface(z=z, x=x, y=y)])
fig_surface.update_layout(title='3D Surface Plot', autosize=False,
width=500, height=500,
margin=dict(l=65, r=50, b=65, t=90))
# Violin Plot
np.random.seed(1)
x_data = ['Apples', 'Oranges', 'Bananas', 'Pears']
y_data = [np.random.randn(200) + i for i in range(len(x_data))]
fig_violin = go.Figure()
for xd, yd in zip(x_data, y_data):
fig_violin.add_trace(go.Violin(x=[xd] * len(yd),
y=yd,
name=xd,
box_visible=True,
meanline_visible=True))
fig_violin.update_layout(title='Violin Plot', yaxis_zeroline=False)
# Show all figures
fig_sankey.show()
fig_radar.show()
fig_treemap.show()
fig_sunburst.show()
fig_parcoords.show()
fig_surface.show()
fig_violin.show()
สำหรับ Basic plots สามารถดูได้ที่
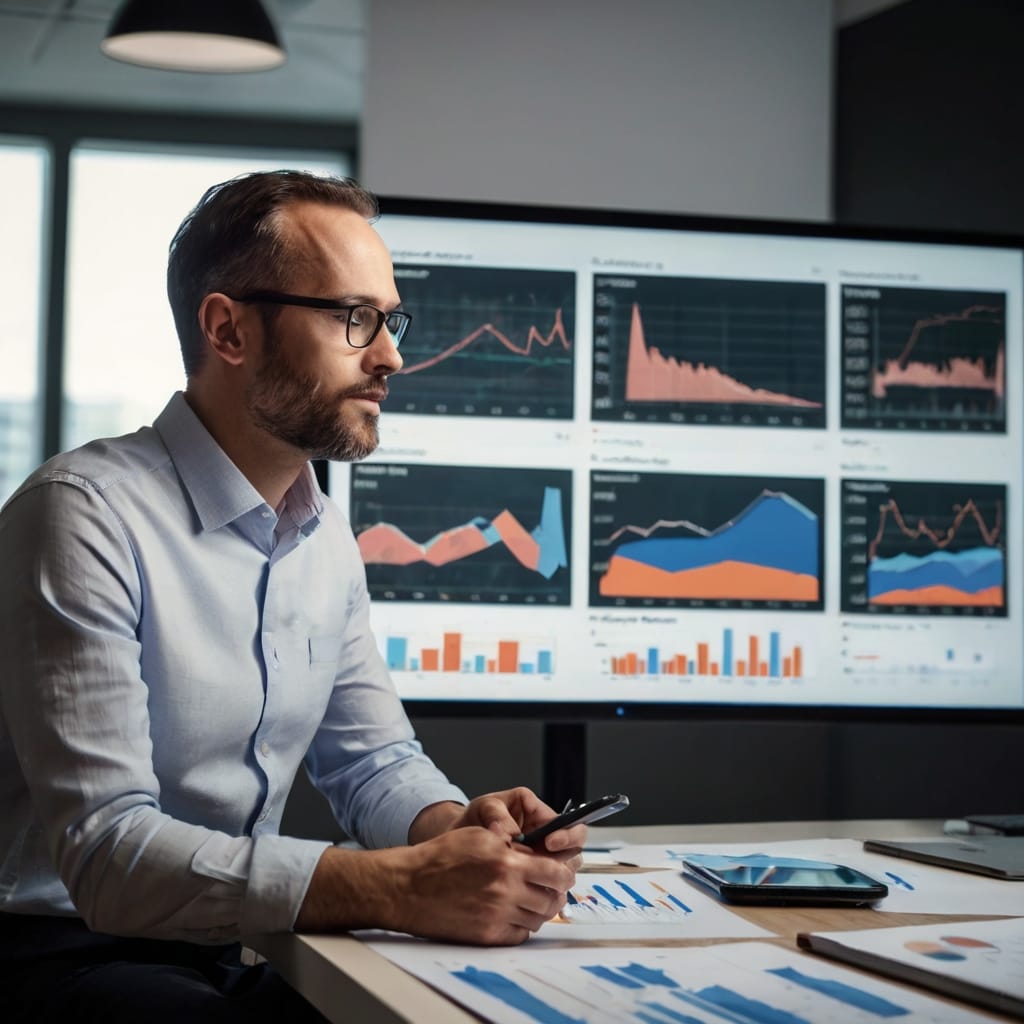
Blog นี้ เขียนร่วมกับ Claude.ai โดยใช้ Prompt
Please explain advanced plots (e.g. Sankey, Radar) using Plotly with sample codes.